Hey, developers welcome to Day 47 of our 90Days 90Projects challenge. And in Day 47 we are going to create a Responsive Accordion using JavaScript.
So to run this code you just need to copy the HTML and CSS code and run it into your code Editor.
Preview
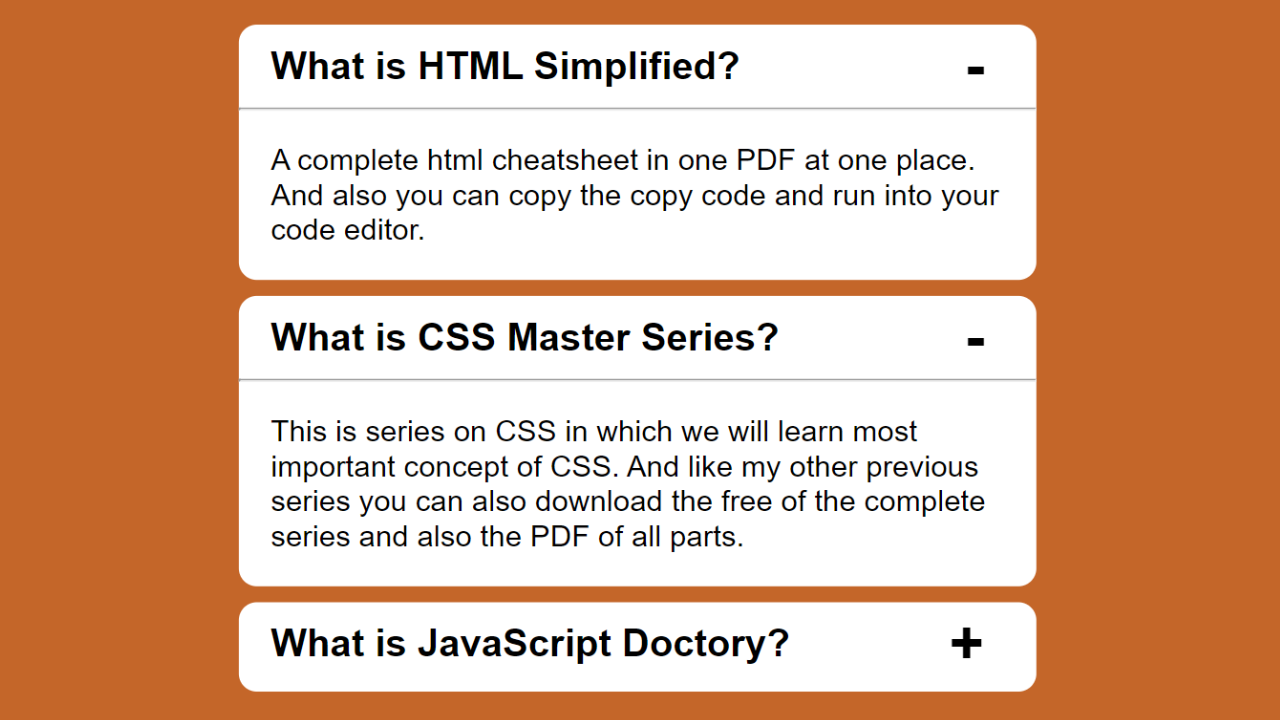
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title> Responsive Accordion using JavaScript </title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="accordion-container">
<div class="accordion">
<div class="accordionTop">
<h2> What is HTML Simplified? </h2>
</div>
<hr>
<div class="content">
<p class="para">
A complete html cheatsheet in one PDF at one place. And also you can copy the copy code and run into your code editor.
</p>
</div>
</div>
<div class="accordion">
<div class="accordionTop">
<h2> What is CSS Master Series? </h2>
</div>
<hr>
<div class="content">
<p class="para">
This is series on CSS in which we will learn most important concept of CSS. And like my other previous series you can also download the free of the complete series and also the PDF of all parts.
</p>
</div>
</div>
<div class="accordion">
<div class="accordionTop">
<h2> What is JavaScript Doctory? </h2>
</div>
<hr>
<div class="content">
<p class="para">
This is the pdf of JavaScript Doctory Series by raju_webdev and in this pdf you will get the javascript topics with example and javascript project ideas.
</p>
</div>
</div>
</div>
</body>
<script src="script.js"></script>
</html>
CSS Code
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: sans-serif;
}
body {
width: 100%;
height: 100vh;
color: white;
display: flex;
align-items: center;
justify-content: center;
background-color: #c46629;
}
.accordion {
color: #000;
background: #fff;
width: 25rem;
height: 2.8rem;
cursor: pointer;
margin: 1rem 0;
overflow: hidden;
border-radius: 9px;
transition: all 0.3s;
}
.accordion:hover {
box-shadow: 0 1px 10px rgb(0, 0, 0, 0.5);
}
.accordionTop {
position: relative;
margin: 0.6rem 1rem;
}
.accordion h2 {
font-size: 1.2rem;
}
.accordionTop::after {
content: '+';
font-weight: bold;
font-size: 1.9rem;
position: absolute;
cursor: pointer;
right: 10px;
top: 50%;
transform: translateY(-50%);
}
.content {
height: 0px;
padding: 1rem;
transition: all 0.3s;
}
hr {
opacity: 0;
}
.accordion.active {
height: auto;
}
.para {
font-size: 15px;
}
.accordion.active hr {
opacity: 1;
}
.accordion.active .accordionTop::after {
content: '-';
}
.accordion.active .content {
height: auto;
transition: all 0.3s;
}
@media screen and (max-width: 420px) {
.accordion {
width: 90%;
margin: 0.6rem auto;
}
.accordionTop h2 {
font-size: 1.1rem;
}
}
JavaScript
const collapse = document.querySelectorAll('.accordionTop')
const myAccordion = document.querySelector('.accordion');
Array.from(collapse).forEach(function (e) {
e.addEventListener('click', function (e) {
myAccordion.style.transition = "all 0.3s";
const current = e.target.parentElement;
current.classList.toggle('active');
})
})