Hey, developers welcome to Day 80 of our 90Days 90Projects challenge. And today in this challenge we are going to Create Laptop Battery Status Detector using JavaScript.
So to run this code you just need to copy the HTML and CSS code and run it into your code Editor.
Preview
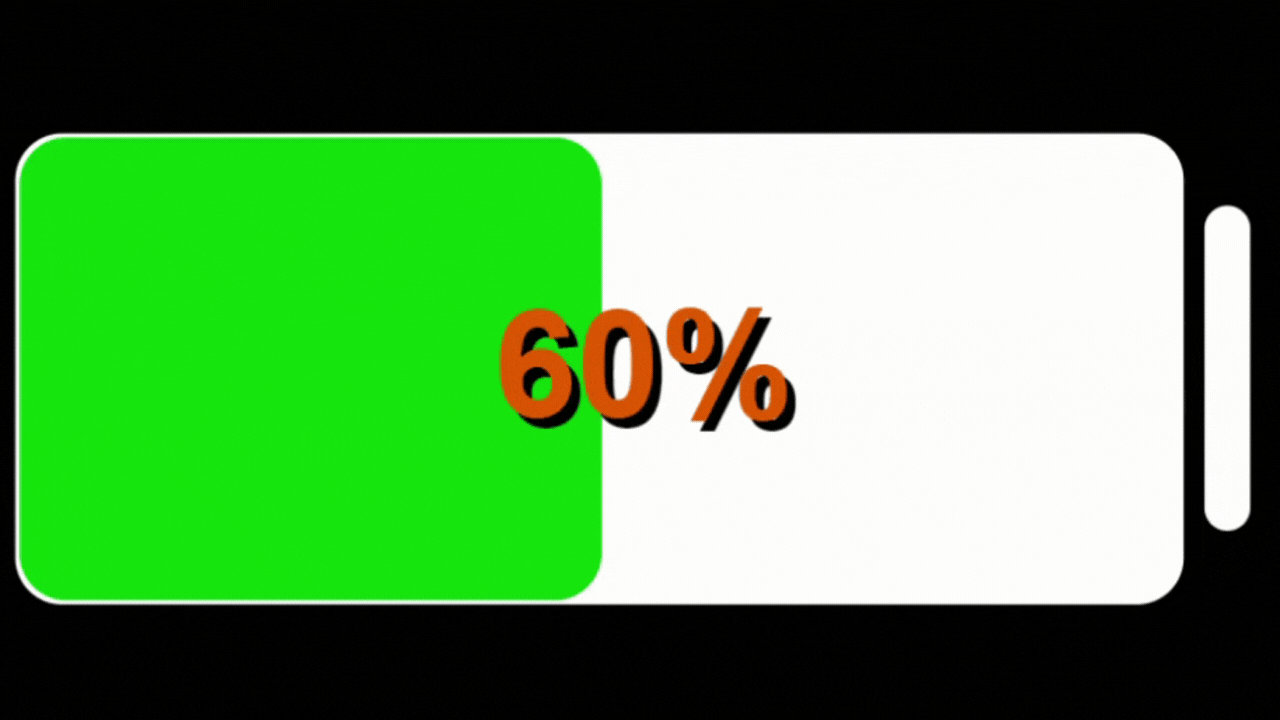
HTML Code
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title> Laptop Battery </title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<div class="main-container">
<div class="battery" id="battery">
<div id="batter-per"></div>
<div class="status"></div>
<p id="batteryStatus"></p>
<div class="sub-container"></div>
</div>
</div>
</div>
</body>
<script src="./batteryScript.js"></script>
</html>
CSS Code
style.css
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
background-color: black;
}
.container {
padding: 10px;
}
.main-container {
padding: 6px;
border-radius: 10px;
display: inline-block;
}
.battery {
width: 250px;
padding: 10px;
border: 1px solid white;
height: 100px;
border-radius: 10px;
background-color: #fff;
position: relative;
}
#batter-per {
position: absolute;
top: 0;
left: 0;
width: 50%;
height: 100%;
background-color: rgb(8, 198, 11);
border-radius: 10px;
animation-name: battery;
animation-iteration-count: 1;
animation-duration: 2s;
}
@keyframes battery {
from {
width: 0%;
}
to {
width: 100%;
}
}
#batteryStatus {
position: relative;
color: #000000;
z-index: 20;
top: 50%;
left: 40%;
font-family: sans-serif;
font-weight: 600;
color: #e65b00;
text-shadow: 2px 2px black;
transform: translateY(-50%);
font-size: 2rem;
}
.sub-container {
background-color: #fff;
height: 70%;
border-radius: 10px;
width: 10px;
position: absolute;
right: -15px;
top: 50%;
transform: translateY(-50%);
}
JavaScript
batteryScript.js
const battery = document.querySelector('#batteryStatus');
let batteryPromise = navigator.getBattery();
batteryPromise.then(batteryFunction);
function batteryFunction(batteryObject) {
BatteryStatus(batteryObject);
setInterval(() => {
BatteryStatus(batteryObject);
}, 2000);
}
function BatteryStatus(batteryObject) {
battery.textContent = `${batteryObject.level * 100}%`;
if (batteryObject.charging == true) {
battery.textContent = `${batteryObject.level * 100}%`;
}
if (batteryObject.charging == false) {
battery.textContent = `${batteryObject.level * 100}%`;
}
let batteryPercentage = parseInt(battery.textContent);
console.log(batteryPercentage);
const batteryContainer = document.getElementById('batter-per');
batteryContainer.style.width = batteryPercentage;
let newNum = `${newNum[0]}${newNum[1]}`;
batteryContainer.style.width = newNum;
}