What is String in Python?
The string is a collection of characters enclosed within single quotes, double quotes, and triple quotes. We can also say that a Python string is a collection of Unicode characters. In Python, a string can be created by enclosing the character or collection of characters in the quotes. A string is the most popular data type in Python.
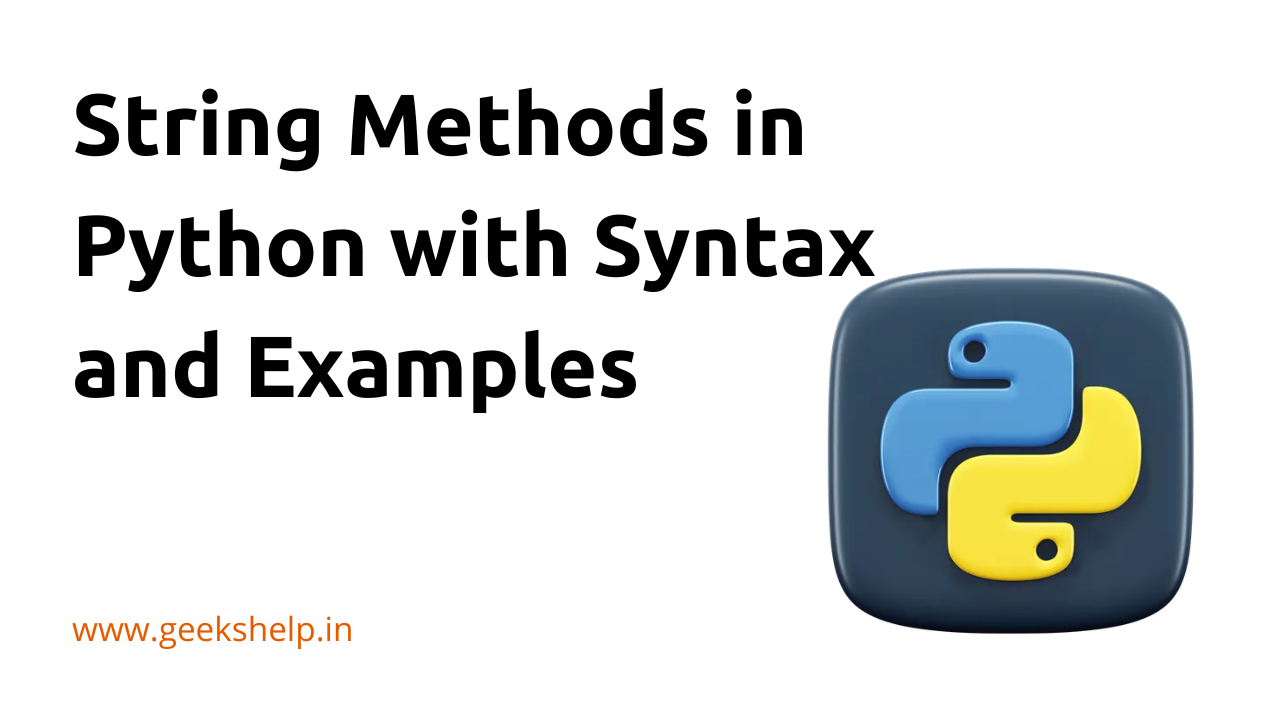
Syntax
Python String Methods
Python has a collection of built-in methods that you can use for strings. All string methods return new values and they don't change the original string. Now let's see all the Python String Methods with Syntax and Examples
1. capitalize()
This method will convert the first character of a string to upper case.
2. casefold()
This method is used to convert the string into lowercase.
3. center()
This Python string method returns a centered string.
4. encode()
This Python string method returns an encoded version of the string.
5. endswith()
This string method will return true if the given string ends with the specified value.
6. expandtabs()
This Python string method will set the tab size of the string.
7. find()
This method searches the string for a specified value and it will return the position of where it was found.
8. format()
This method in Python string is used to format specified value in a string.
9. isalnum()
This Python string method will return true if all the characters in the string are alphanumeric.
10. isalpha()
This Python string method will return true if all the characters in the string are alphabet.
11. isascii()
This Python string method will return true if all the characters in the string are ascii characters.
12. isdecimal()
This Python string method will return true if all the characters in the string are decimals.
13. isdigit()
This Python string method will return true if all the characterscter in the string are digit.
12. isidentifier()
This Python string method will return true if the string is an identifier.
13. islower()
This Python string method will return true if all the characters in the string are lowercase.
14. isnumeric()
This Python string method will return true if all the characters in the string are numeric.
15. isprintable()
This Python string method will return true if all the characters in the string are printable.
16. isspace()
This Python string method will return true if all the characters in the string are whitespaces.
17. isupper()
This Python string method will return true if all the characters in the string are upper case.
18. join()
This string method will convert the elements of an iterable into a string.
19. ljust()
This Python string method will return a left-justified version of the string.
20. lower()
This Python string method will convert a string into lower case.
21. lstrip()
This Python string method will return a left-trim version of the string.
22. replace()
This string method will return a string where the specified value is replaced with a specified value.
23. rstrip()
This Python string method will returns a right-trim version of a string.
24. split()
This Python string method will splits the string at the specified separator and return a list.
25. startswith()
This string method will return true if the given string starts with the specified value.
26. strip()
This method will return a trimmed version of the string.
27. title()
This Python string method will convert the first character of each word to upper case.
28. upper()
This string method will convert a string into upper case.
29. translate()
Modify the string according to the translation mappings provided.
30. zfill()
This Python string method will return a copy of the string with '0' characters padded at the beginning to the string.
31. swapcase()
This Python string method will convert all uppercase characters to lowercase and vice versa.
32. istitle()
This Python string method will return true if the string is titled properly otherwise it will return false.
33. len()
This Python string method will return the length of a string.